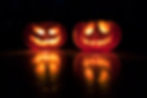
It's almost Halloween as I write this. What's better than to have fun with coding by writing a Halloween animation with Python? I'll tell you what's better: writing a tutorial about it.
Let's get started. Here's the animation you'll create in this tutorial:
Setting Up the Halloween Animation
You'll use the turtle module for this animation. This module is a perfect one to quickly create graphics without too much fuss. If you've never used the turtle module in Python before, don't worry as I'll explain each step as I go along.
I'll assume you're using Python installed on your computer. If you're using a web-based platform, you may need to adjust some of the values to make your animation fit in your display window.
You can start by importing turtle and creating a Screen and a couple of Turtles:
import turtle
window = turtle.Screen()
window.bgcolor("black")
# Whole pumpkin
pumpkin = turtle.Turtle()
pumpkin.hideturtle()
pumpkin.color("orange")
pumpkin.dot(200)
# The turtle to "carve" the pumpkin
carver = turtle.Turtle()
turtle.done()
One of the Turtles represents the whole pumpkin which is orange, of course! It creates a big orange dot. You'll then need another turtle to 'carve' this pumpkin. The methods from the turtle module used here are fairly self-explanatory:
turtle.Screen() and turtle.Turtle() creates instances of these classes
The Screen method bgcolor() stands for background colour
The Turtle methods color() and dot() choose the colour and draw a dot
hideturtle() simply hides the drawing pen so that all you see is the drawing itself. You're using it for the pumpkin Turtle but not for the carver one. So you'll see a small black arrow representing the carver Turtle
Pumpkins are not spheres, but let's not be too fussy. We can flatten the lower part of the pumpkin by drawing a thick, black line to cover the lower part of the orange circle. The method penup() allows you to move the Turtle without drawing a line and you can guess what pendown() does. There are different ways of moving the Turtle, too. Here you're using setposition() to place the Turtle at a particular set of coordinates and forward to move the Turtle in the direction it's facing:
import turtle
window = turtle.Screen()
window.bgcolor("black")
# Whole pumpkin
pumpkin = turtle.Turtle()
pumpkin.hideturtle()
pumpkin.color("orange")
pumpkin.dot(200)
# The turtle to "carve" the pumpkin
carver = turtle.Turtle()
# "Flatten" the lower part of the pumpkin
carver.penup()
carver.setposition(-200, -100)
carver.pensize(60)
carver.pendown()
carver.forward(600)
carver.pensize(2)
turtle.done()
Carving the Pumpkin
Next step: you need to start carving the pumpkin. Luckily, this is a less messy process in Python than in real life! And if you make a mistake, you can undo it, unlike in the real world.
You can create a function to carve the pumpkin. You'll see a bit later why we're using a function for the carving process:
import turtle
window = turtle.Screen()
window.bgcolor("black")
# Whole pumpkin
pumpkin = turtle.Turtle()
pumpkin.hideturtle()
pumpkin.color("orange")
pumpkin.dot(200)
# The turtle to "carve" the pumpkin
carver = turtle.Turtle()
# "Flatten" the lower part of the pumpkin
carver.penup()
carver.setposition(-200, -100)
carver.pensize(60)
carver.pendown()
carver.forward(600)
carver.pensize(2)
def carve_pumpkin(colour):
carver.color(colour)
# Make eyes
def make_eye(start, orientation):
carver.setheading(0)
carver.penup()
carver.setposition(*start)
carver.pendown()
carver.begin_fill()
carver.forward(orientation * 40)
carver.setheading(orientation * 135)
carver.forward(orientation * 70)
carver.end_fill()
make_eye((-50, 20), 1)
make_eye((50, 20), -1)
carve_pumpkin("black")
turtle.done()
The function carve_pumpkin(colour) takes an argument. This will allow you to choose what colour you want the holes to be. You'll use this later when you make the pumpkin light up and flash!
Since you're making two eyes, it's easier to create a function inside carve_pumpkin() to create an eye. The input parameters of make_eye() are start and orientation. These parameters allow you to fine tune where the drawing of the eye should start, and whether this is a right eye or a left eye:
start needs to be a tuple or a list containing two numbers. These are the x- and y- coordinates of the starting point. You'll spot the use of the unpacking operator in the make_eye() function in carver.setposition(*start). If you're not familiar with this operator, you can look it up, but in brief it opens up the tuple or list and puts its contents instead. Don't worry too much about this!
orientation is either 1 or -1 to create symmetrical images.
There's a new method from the turtle module you use here. This is setheading() which needs an angle and it turns the Turtle to head towards this angle.
You can then call make_eye() twice, once for each eye.
Making the Mouth and Nose
Next, you need to add the mouth and nose. You can customise your own mouth and nose for your pumpkin, if you prefer, but here are my versions for the mouth and nose:
import turtle
window = turtle.Screen()
window.bgcolor("black")
# Whole pumpkin
pumpkin = turtle.Turtle()
pumpkin.hideturtle()
pumpkin.color("orange")
pumpkin.dot(200)
# The turtle to "carve" the pumpkin
carver = turtle.Turtle()
# "Flatten" the lower part of the pumpkin
carver.penup()
carver.setposition(-200, -100)
carver.pensize(60)
carver.pendown()
carver.forward(600)
carver.pensize(2)
def carve_pumpkin(colour):
carver.color(colour)
# Make eyes
def make_eye(start, orientation):
carver.setheading(0)
carver.penup()
carver.setposition(*start)
carver.pendown()
carver.begin_fill()
carver.forward(orientation * 40)
carver.setheading(orientation * 135)
carver.forward(orientation * 70)
carver.end_fill()
make_eye((-50, 20), 1)
make_eye((50, 20), -1)
# Make mouth
carver.penup()
carver.setposition(-50, -30)
carver.setheading(45)
carver.pendown()
carver.pensize(1)
carver.begin_fill()
for _ in range(5):
carver.forward(15)
carver.right(90)
carver.forward(15)
carver.left(90)
carver.setheading(260)
carver.forward(20)
carver.setheading(180)
carver.forward(99)
carver.end_fill()
# Make nose
carver.penup()
carver.setposition(0, 0)
carver.setheading(90)
carver.shape("triangle")
carver.stamp()
carve_pumpkin("black")
turtle.done()
There are a few more turtle methods here:
begin_fill() and end_fill() can be used to 'sandwich' code that draws a shape. This shape is then filled in using the current colour of the turtle.
right() and left() turn the Turtle by the angle given as an argument in these methods.
shape() changes the shape of the drawing pen on the screen. There aren't many options, but "triangle" is one of them
stamp() creates a permanent copy (or stamp) of the turtle shape which will remain in place even when you move the turtle. This makes it easy to create the nose as a triangle.
The pumpkin is ready. But there's more you can do to the animation.
Writing Text on the Animation Screen
You want to wish Happy Halloween to anyone who sees your Halloween animation in Python. You can write text on your animation using the write() method in the turtle module:
import turtle
window = turtle.Screen()
window.bgcolor("black")
# Whole pumpkin
pumpkin = turtle.Turtle()
pumpkin.hideturtle()
pumpkin.color("orange")
pumpkin.dot(200)
# The turtle to "carve" the pumpkin
carver = turtle.Turtle()
# "Flatten" the lower part of the pumpkin
carver.penup()
carver.setposition(-200, -100)
carver.pensize(60)
carver.pendown()
carver.forward(600)
carver.pensize(2)
def carve_pumpkin(colour):
carver.color(colour)
# Make eyes
def make_eye(start, orientation):
carver.setheading(0)
carver.penup()
carver.setposition(*start)
carver.pendown()
carver.begin_fill()
carver.forward(orientation * 40)
carver.setheading(orientation * 135)
carver.forward(orientation * 70)
carver.end_fill()
make_eye((-50, 20), 1)
make_eye((50, 20), -1)
# Make mouth
carver.penup()
carver.setposition(-50, -30)
carver.setheading(45)
carver.pendown()
carver.pensize(1)
carver.begin_fill()
for _ in range(5):
carver.forward(15)
carver.right(90)
carver.forward(15)
carver.left(90)
carver.setheading(260)
carver.forward(20)
carver.setheading(180)
carver.forward(99)
carver.end_fill()
# Make nose
carver.penup()
carver.setposition(0, 0)
carver.setheading(90)
carver.shape("triangle")
carver.stamp()
carve_pumpkin("black")
# Write text on animation
text = turtle.Turtle()
text.hideturtle()
text.color("orange")
text.penup()
text.sety(175)
text.write("Happy Halloween", font=("Trattatello", 60, "bold"), align="center")
turtle.done()
You've created a new Turtle and also made it orange to remain on theme! The sety() method is similar to setposition() but only sets the y-coordinate. The write() method takes a font parameter which allows you to customise how the text looks.
NOTE that the font I'm using may not work on your system. You can replace this with any font of your choice that works on your system!
Flashing Lights!
Finally, time to make your Halloween animation in Python flash as if it has a flickering candle inside, or in reality a flashing light bulb!
You'll use the time module which you need to import at the top and you'll use time.time() to start a timer which resets every one second. You also alternate the colours between yellow and black and use these colours to call the carve_pumpkin(colour) you defined earlier:
import turtle
import time
window = turtle.Screen()
window.bgcolor("black")
# Whole pumpkin
pumpkin = turtle.Turtle()
pumpkin.hideturtle()
pumpkin.color("orange")
pumpkin.dot(200)
# The turtle to "carve" the pumpkin
carver = turtle.Turtle()
# "Flatten" the lower part of the pumpkin
carver.penup()
carver.setposition(-200, -100)
carver.pensize(60)
carver.pendown()
carver.forward(600)
carver.pensize(2)
def carve_pumpkin(colour):
carver.color(colour)
# Make eyes
def make_eye(start, orientation):
carver.setheading(0)
carver.penup()
carver.setposition(*start)
carver.pendown()
carver.begin_fill()
carver.forward(orientation * 40)
carver.setheading(orientation * 135)
carver.forward(orientation * 70)
carver.end_fill()
make_eye((-50, 20), 1)
make_eye((50, 20), -1)
# Make mouth
carver.penup()
carver.setposition(-50, -30)
carver.setheading(45)
carver.pendown()
carver.pensize(1)
carver.begin_fill()
for _ in range(5):
carver.forward(15)
carver.right(90)
carver.forward(15)
carver.left(90)
carver.setheading(260)
carver.forward(20)
carver.setheading(180)
carver.forward(99)
carver.end_fill()
# Make nose
carver.penup()
carver.setposition(0, 0)
carver.setheading(90)
carver.shape("triangle")
carver.stamp()
carve_pumpkin("black")
# Write text on animation
text = turtle.Turtle()
text.hideturtle()
text.color("orange")
text.penup()
text.sety(175)
text.write("Happy Halloween", font=("Trattatello", 60, "bold"), align="center")
window.tracer(0)
start = time.time()
count = 0
colours = "yellow", "black"
while True:
if time.time() - start > 1:
carve_pumpkin(colours[count % 2])
window.update()
count += 1
start = time.time() # Reset timer
turtle.done()
You're also using the tracer() and update() methods here, also from the turtle module. They are methods you can use on your Screen object. These allow you to draw the shapes you need instantly and not have to wait for the turtle to make its way across the screen, drawing things gradually. The variable colours is a tuple, but you can use a list too if you prefer.
The % operator returns the remainder when two numbers are divided. So using count % 2 is a good way of checking whether count is even or odd and will always return either 0 or 1, since those are the only two remainders possible when you divide by 2. This allows you to swap between yellow and black and then back to yellow, and so on.
And you're done. You now have a Halloween animation in Python which you've written using the turtle module. Can you add your own features to this animation now? Or maybe you are ready to draw a Christmas tree in Python.
Enjoyed this and want to learn more Python for free? Check out our sister site The Python Coding Book, for in-depth guides on all things Python, or follow us on Twitter @codetoday_ and @s_gruppetta_ct